Table Of Content
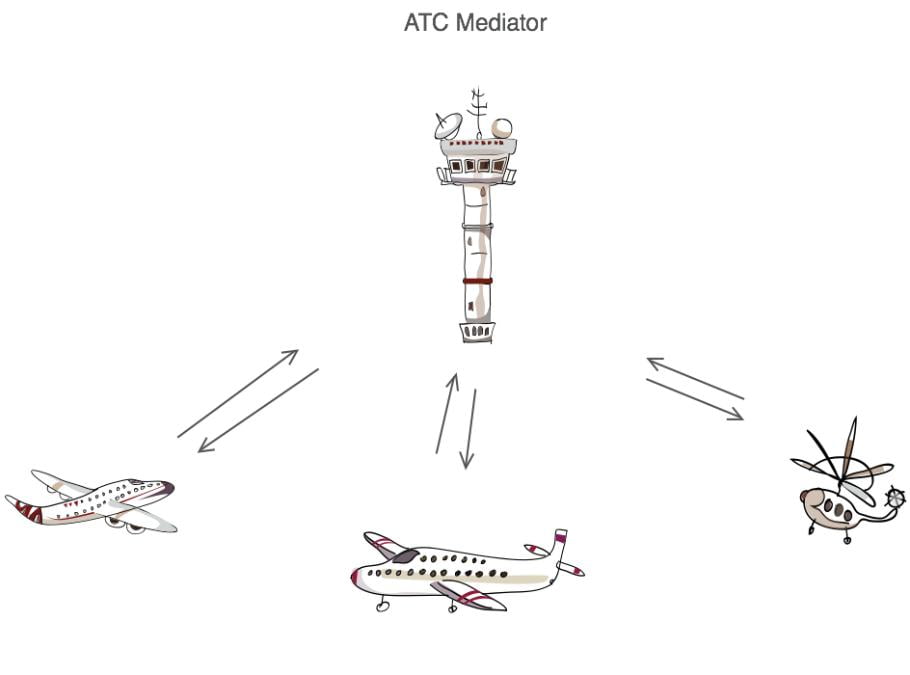
The Chatroom is the central hub through which all communication takes place. At this point only one-to-one communication is implemented in the Chatroom, but would be trivial to change to one-to-many. By implementing this logic directly in the form element classes, it becomes much harder to reuse these classes in other forms. The users within the chatroom won’t talk to each other directly. Instead, the chatroom serves as the mediator between the users. You can compare this pattern to the relationship between an air traffic controller and a pilot.
Code examples
It makes the communication between objects encapsulated with a mediator object. Objects don’t communicate directly with each other, but instead, they communicate through the mediator. The essence of the mediator pattern is to "define an object that encapsulates how a set of objects interact". If the objects interact directly with one other, the system components will be strongly connected with each other, resulting in increased maintainability costs and ease of extension. The Mediator pattern focuses on acting as a bridge between items to facilitate communication and aid in the implementation of lose-coupling between components.
Mediator pattern
Mediator interfaces define methods of communication with components, including the notification method. This way, the Mediator pattern lets you encapsulate a complex web of relations between various objects inside a single mediator object. The fewer dependencies a class has, the easier it becomes to modify, extend or reuse that class.
UML class and sequence diagram
So, create an abstract class named User.cs and copy and paste the following code. This abstract class has two abstract methods, i.e., Send and Receive. The pattern lets you extract all the relationships between classes into a separate class, isolating any changes to a specific component from the rest of the components.
This is a perfect place to use Strategy and swap path creation algorithms in runtime. This way, we avoid the mutability of Strategy and make the program a bit easier to debug, but we are now forced to pass in Strategy each time we want to doSomething . We have a Strategy interface with an algorithm function that ConcreteStrategies implement. There’s a clear separation between Strategies and Context that is aware of the current algorithm. Also I've used mediator before to communicate among very dispar systems, and legacy frameworks.
Real world example of mediator pattern
A pattern-based approach to protocol mediation for web services composition - ScienceDirect.com
A pattern-based approach to protocol mediation for web services composition.
Posted: Thu, 28 Dec 2017 11:27:51 GMT [source]
Create a class file named ConcreteUser.cs and copy and paste the following code. This is a concrete class that implements the User abstract class and provides the implementation for the Send and Receive abstract methods. The Send method sends a message to a particular Facebook group.
A yet another article about MediatR and CQRS in .Net Core
Instead, they speak to an air traffic controller, who sits in a tall tower somewhere near the airstrip. Without the air traffic controller, pilots would need to be aware of every plane in the vicinity of the airport, discussing landing priorities with a committee of dozens of other pilots. Misuse of a mediator can result in crippling the interfaces of the mediator's colleague classes. We will next move ahead and create the Colleague interface and the implementation classes.
In the worst case, imagine that the battle tactics change so that both the soldier and sniper units can now attack simultaneously. We can address such challenges, similarly as done in real life. Place a Commander in a base camp that will act as the mediator.
Design and Implementation of a DDD-Based Modular Monolith - InfoQ.com
Design and Implementation of a DDD-Based Modular Monolith.
Posted: Fri, 27 Sep 2019 07:00:00 GMT [source]
It is mainly because of its ability to encapsulate communication logic between sets of objects to fulfill some business requirements. A disadvantage of this pattern often being discussed in the developer community is that the mediator object can gradually get inflated and finally become overly complex. But, by following SOLID design principles, specifically the Single Responsibility principle, you can segregate the responsibilities of a mediator into separate classes.
Instead, the participant only needs to check the captioning system to understand a message and then speak without knowledge of the words and their meanings used by the other attendees. In this example, considering a general scenario, the person hosting the meeting would have to learn every language and find a way to simultaneously communicate with each other. However, the Mediator pattern helps eliminate mutual dependencies between the different people on the call.
By implementing this pattern, we can create a bidirectional communication layer, which can be used by a class in order to interact with others through a common object acting as a mediator. With the mediator pattern, communication between objects is encapsulated within a mediator object. Objects no longer communicate directly with each other, but instead communicate through the mediator. This reduces the dependencies between communicating objects, thereby reducing coupling.
In the mediator design pattern, the objects don’t communicate with one another directly but through the mediator. When an object needs to communicate with another object or a set of objects, it transmits the message to the mediator. The mediator then transmits the message to each receiver object in a form that is understandable to it. Mediator is a behavioral design pattern that lets you reduce chaotic dependencies between objects. The pattern restricts direct communications between the objects and forces them to collaborate only via a mediator object. The Mediator pattern is considered one of the most important and widely adopted patterns.